Error
Handling enables programmers to write clearer, more robust, more fault-tolerant
programs. Error handling enables the programmer to attempt to recover (i.e.,
continue executing) from infrequent fatal errors rather than letting them occur
and suffering the consequences (such as loss of application data). If an error
is severe and recovery is not possible, the program can be exited
"gracefully"-all files can be closed and notification can be given
that the program is terminating. The recovery code is called an error handler.
Error
handling is designed for dealing with synchronous errors such as an attempt to
divide by 0 (that occurs as the program executes the divide instruction). Other
common examples of synchronous errors are memory exhaustion, an out-of-bound
array index, and arithmetic overflow. Error handling provides the programmer
with a disciplined set of capabilities for dealing with these types of errors.
Error
Categories
There are
three main types of errors that could occur while your application is being
used:
- Syntax Errors: A syntax error comes from your mistyping a word or forming a bad expression in your code. It could be that you misspelled a keyword such as ByVel instead of ByVal. It could also be a bad expression. Examples are:524+ + 62.55
- Run-Time Errors: After all syntax errors have been fixed, the program may be ready for the user. The time period a person is using an application is called run-time. There are different types of problems that a user may face when interacting with your program. For example, imagine that, in your code, you indicate that a picture would be loaded and displayed to the user but you forget to ship the picture or the directory of the picture indicated in your code becomes different when a user opens your application. In this case, when you compiled and executed the application in your machine, everything was fine. This is a type of run-time error.Run-time errors are mostly easy to fix because you will know what the problem is occurring and why.
- Logic Errors: These are
errors that don't fit in any of the above categories. They could be caused
by the user misusing your application, a problem with the computer on
which the application is running while the same application is working
fine in another computer. Because logic errors can be
vague, they can also be difficult to fix.
Dealing
with errors at run-time is a two step process:
- Trap the ErrorBefore you can deal with an error, you need to know about it. You use VB's On Error statement to setup an error trap.
- Handle the ErrorCode in your error handler may correct an error, ignore it, inform the user of the problem, or deal with it in some other way. You can examine the properties of the Err object to determine the nature of the error. Once the error has been dealt with, you use the Resume statement to return control to the regular flow of the code in the application.
Trapping
Errors at Run-Time
Before you can do anything
to deal with a run-time error, you need to capture the error. You use the On
Error statement to enable an error trap. On Error will redirect the execution
in the event of a run-time error. There are several forms of the On Error
statement:
- On Error Goto labelThis form of the On Error statement redirects program execution to the line label specified. When you use this form of On Error, a block of error handling code is constructed following the label.
- On Error Resume NextThis form of the On Error statement tells VB to continue with the line of code following the line where the error occurred. With this type of error trap, you would normally test for an error at selected points in the program code where you anticipate that an error may occur.
- On Error Goto 0On Error Goto 0 disables any error handler within the current procedure. A run-time error that occurs when no error handler is enabled or after an On Error Goto 0 is encountered will be handled using VB's default error handling logic.
Example : Division by Zero
Private Sub
CmdCalculate_Click()
Dim firstNum, secondNum As
Double
firstNum = Txt_FirstNumber.Text
secondNum = Txt_SecondNumber.Text
On Error GoTo error_handler
Lbl_Answer.Caption = firstNum / secondNum
Exit Sub
'To prevent error handling even the inputs are valid
error_handler:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You attempt to divide a number by zero!Try
again!"
End Sub
Private Sub Txt_FirstNumber_GotFocus()
Lbl_ErrorMsg.Visible
= False
End Sub
Private Sub
Txt_SecondNumber_GotFocus()
Lbl_ErrorMsg.Visible
= False
End Sub

Building Error Handlers
1. Trapping
an error using the On Error statement is only the first step in dealing with
run-time errors in your code. You must also deal with the error in some way,
even if the error handling code is as simple as ignoring the error (a perfectly
valid approach in some situations) or displaying a message for the user.
2. The first
step in handling an error is determining the nature of the error. This is
accomplished by examining the properties of Visual Basic's Err object. The Err
object includes the following properties:
·
Number
This is the error number that was raised.
·
Description
This contains a descriptive message about the error. Depending on the error,
the description may or may not be useful. (Microsoft Access, for example, has
the the infamous error message "There is no message for this error.")
·
Source
The Source property tells you what object generated the error.
·
HelpContext
If a help file has been defined for the component that raised the error, this
property will give you the help context ID. You can use this property along
with the HelpFile property to display context sensitive help for errors in your
application or as a debugging aid.
·
HelpFile
This is the name of the help file that contains additional information about
the error (if a help file has been provided).
3. Once you
have trapped and handled the error, you need to tell Visual Basic where to
continue with program execution. There are several options available when an
error handling block is entered using On Error Goto label:
·
Resume
The Resume statement tells VB to continue execution with the line that
generated the error.
·
Resume Next
Resume Next instructs Visual Basic to continue execution with the line
following the line that generated the error. This allows you to skip the
offending code.
·
Resume label
This
allows you to redirect execution to any label within the current procedure. The
label may be a location that contains special code to handle the error, an exit
point that performs clean up operations, or any other point you choose.
·
Exit
You
can use Exit Sub, Exit Function, or Exit Property to break out of the current
procedure and continue execution at whatever point you were at when the
procedure was called.
·
End
This
is not recommended, but you can use the End statement to immediately terminate
your application. Remember that if you use End, your application is forcibly
terminated. No Unload, QueryUnload, or Terminate event procedures will be
fired. This is the coding equivalent of a gunshot to the head for your
application.
Example: Nested Error Handling Procedure
Private Sub CmdCalculate_Click()
Dim firstNum, secondNum As
Double
On Error GoTo error_handler1
firstNum = Txt_FirstNumber.Text
secondNum = Txt_SecondNumber.Text
On Error GoTo error_handler2
Lbl_Answer.Caption = firstNum / secondNum
Exit Sub 'To prevent errror handling even the inputs are valid
error_handler2:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You attempt to divide a number by zero!Try
again!"
Exit Sub
error_handler1:
Lbl_Answer.Caption = "Error"
Lbl_ErrorMsg.Visible = True
Lbl_ErrorMsg.Caption = " You are not entering a number! Try again!"
End Sub
Private Sub Txt_FirstNumber_GotFocus()
Lbl_ErrorMsg.Visible = False
End Sub
Private Sub Txt_SecondNumber_GotFocus()
Lbl_ErrorMsg.Visible = False
End Sub
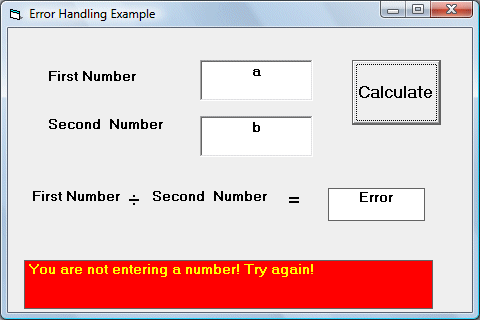